8.3 The Wrapper Classes
Wrapper classes were introduced with the discussion of the primitive data types (Table 2.16, p. 43), and also in connection with boxing and unboxing of primitive values (§2.3, p. 45). Primitive values in Java are not objects. To manipulate these values as objects, the java.lang package provides a wrapper class for each of the primitive data types (shown in the bottom left of Figure 8.2). The name of the wrapper class is the name of the primitive data type with an uppercase letter, except for int (Integer) and char (Character). All wrapper classes are final, meaning that they cannot be extended. The objects of all wrapper classes that can be instantiated are immutable; in other words, the value in the wrapper object cannot be changed.
Although the Void class is considered a wrapper class, it does not wrap any primitive value and is not instantiable (i.e., has no public constructors). It just denotes the Class object representing the keyword void. The Void class will not be discussed further in this section.
In addition to the methods defined for constructing and manipulating objects of primitive values, the wrapper classes define useful constants, fields, and conversion methods.
A very important thing to note about the wrapper classes is that their constructors are now deprecated. So these constructors should not be used to create wrapper objects, as these constructors will be removed from the API in the future. As we shall see later in this section, the APIs include methods for this purpose that provide significantly improved space and time performance.
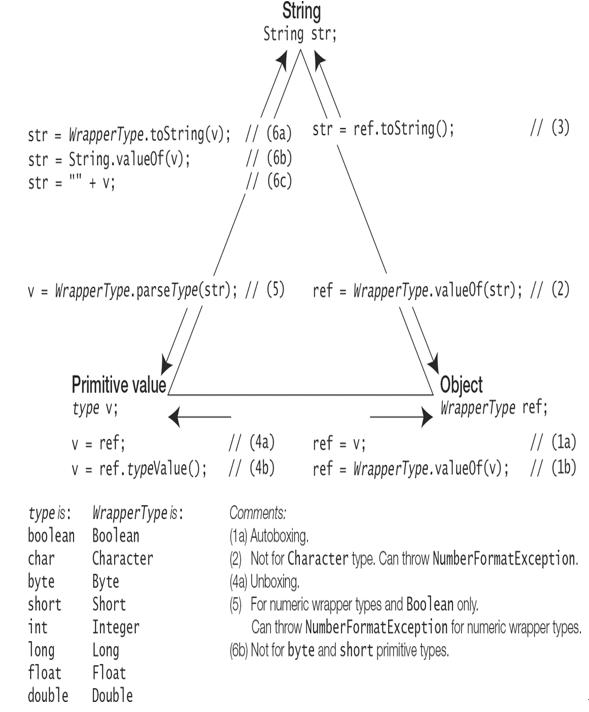
Figure 8.2 Converting Values among Primitive, Wrapper, and String Types
Common Wrapper Class Utility Methods
Converting Primitive Values to Wrapper Objects
Autoboxing is a convenient way to wrap a primitive value in an object ((1a) in Figure 8.2 and §2.3, p. 45).
Character charObj1 = ‘\n’;
Boolean boolObj1 = true;
Integer intObj1 = 2020;
Double doubleObj1 = 3.14;
We can also use the following valueOf(type v) method that takes the primitive value of type to convert as an argument ((1b) in Figure 8.2).
static
WrapperType
valueOf(
type
v)
Character charObj1 = Character.valueOf(‘\n’);
Boolean boolObj1 = Boolean.valueOf(true);
Integer intObj1 = Integer.valueOf(2020);
Double doubleObj1 = Double.valueOf(3.14);
Leave a Reply